The SQL Server NCHAR function is a String method that returns the Unicode character at the specified integer value, as defined in Unicode standards. The syntax of the SQL NCHAR Function is
SELECT NCHAR(Integer_Expression) FROM [Source]
Integer_Expression: Please specify the valid numeric value. If the value is out of the range (something like 123456), it will return NULL as output.
SQL Server NCHAR Function Example
The NCHAR Function returns the Unicode character of the given expression or integer value. The following query may show you multiple ways to use this one.
DECLARE @Str1 NCHAR(25) DECLARE @Str2 NCHAR(5) -- Initialize the variables. SET @Str1 = 'è' SET @Str2 = 'ë' SELECT UNICODE(@Str1) AS Result1, NCHAR(UNICODE(@Str1)) AS Result2, UNICODE(@Str2) AS Result3, NCHAR(UNICODE(@Str2)) AS Result4; SELECT NCHAR(UNICODE('âëz')) AS Result5; SELECT NCHAR(UNICODE('Å28')) AS Result6;
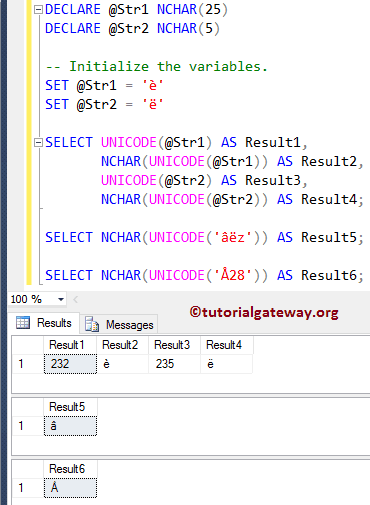
Below lines of SQL code are used to declare two variables of type NCHAR. Next, we assigned the string data è and ë
DECLARE @Str1 NCHAR(25) DECLARE @Str2 NCHAR(5) SET @Str1 = 'è' SET @Str2 = 'ë'
From the below statement, you can see we are finding the UNICODE value of è, ë, and applying the NCHAR function to that Unicode value. We also assigned new names to the result as ‘Result1’ to ‘Result4’ using the ALIAS Column.
SELECT UNICODE(@Str1) AS Result1, NCHAR(UNICODE(@Str1)) AS Result2, UNICODE(@Str2) AS Result3, NCHAR(UNICODE(@Str2)) AS Result4;
In the next line, We used the SQL NCHAR function on a group of Ncharacters (word). Here, the UNICODE method will return the Unicode value of the leftmost character (i.e., â), which should be 226. Next, the NCHAR String method will return the Character at 226, and that is â
SELECT NCHAR(UNICODE('âëz')) AS Result5;
NCHAR Function Example 2
In this example, we will use the SQL Server NCHAR function inside the WHILE LOOP.
I suggest you refer to the UNICODE, SUBSTRING, and WHILE LOOP articles in SQL Server to understand the below code.
DECLARE @i INT, @string1 NCHAR(16) -- Initialize the variables. SET @i = 1 SET @string1 = 'TùtÓrïål GãtÊwáy' WHILE @i <= LEN(@string1) BEGIN SELECT SUBSTRING(@string1, @i, 1) AS [NChar Value], UNICODE(SUBSTRING(@string1, @i, 1)) AS [UNICODE Result], NCHAR(UNICODE(SUBSTRING(@string1, @i, 1))) AS [NCHAR Result] SET @i = @i + 1 END
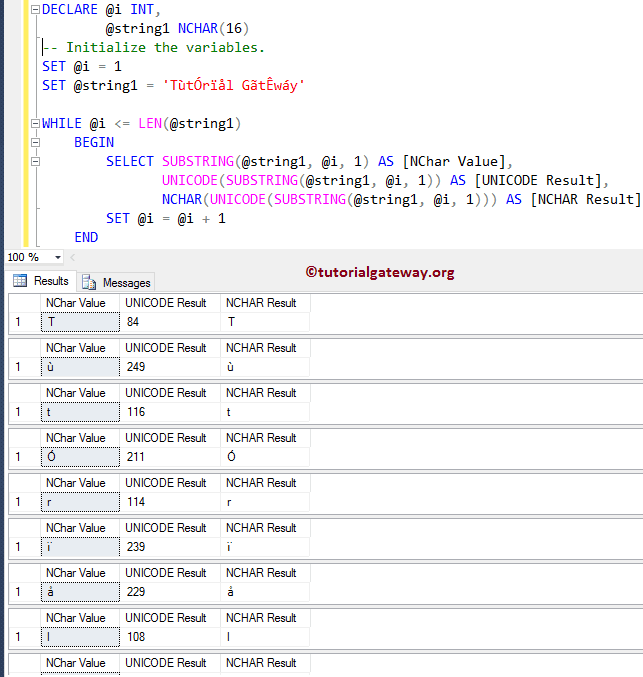